HOW TO CODE IN C++ (TUTORIAL)
CLICK THE LINK AND FOLLOW THE STEPS TO IF YOU WANT TO FOLLOW ALONG WITH THE TUTORIAL: https://www.youtube.com/watch?v=xMHJGd3wwZk
Click this link for the compiler I am coding in (replit): https://replit.com/~
HOW TO MAKE A MADLIBS GAME IN C++
STEP 1: SETUP YOUR FUNCTION AND INCLUDE LIBRARIES
In order to make something in C++, you will need to get all your code from a library. For this tutorial, we will be using the standard library also known as “iostream.” In order to include it, we’ll write #include <iostream>. All libraries have to be used by typing in a little phrase before writing the code. For this library, you have to write std:: and then your code, but we can bypass this. On the line below, write: using namespace std;
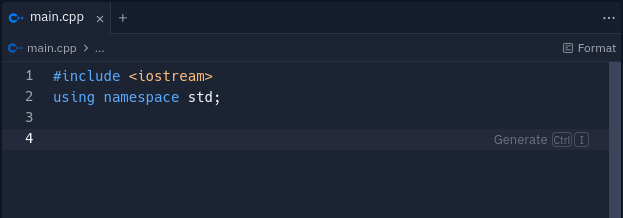
Now let’s make a function to hold all of our code in. A function is used to separate each part of your code, and without it, nothing would work. A function would be written as: int(main) { }. All the code will be held inside the curly brackets, so make sure to press enter when in the curly brackets to make your code easier to read like the example below.
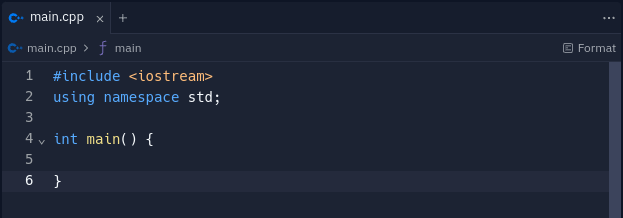
STEP 2: MAKE YOUR MADLIB INPUTS
This step will make it so the program will tell you to put in a noun, adjective, verb, or one of your choice. For this example, I will just use a noun and a verb, but you can create more of these to make your game more fun. First, you want to create a variable, which is what will be holding the data you input. For this you will write string, then the name of your variable (you choose!), and then =. Then you just add a semicolon to end the phrase, making it so the string holds no information.
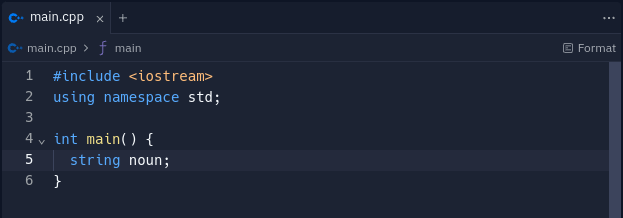
Now you will need to allow the person to input the information, in this case the noun, so that the code will know what you want the noun to be. To do this, we will start with the instructions. Write cout >> “Type a noun: “; to print the instructions in the console. You can press “run” to test your code. For the input part, you can write cin << and then you can put in your variable’s name, in this case name. The full line will look like: cin << name;
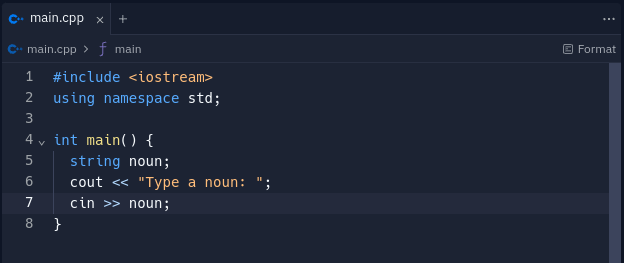
For the next step, I am going to copy and paste (ctrl c / ctrl v) lines 5 – 7 below, and I’m going to change the name of the variable in the string and cin along with adding changing the instructions in the cout. You can make as many of these as you want, but it will make the last step harder if you add too many. I will only make two inputs.
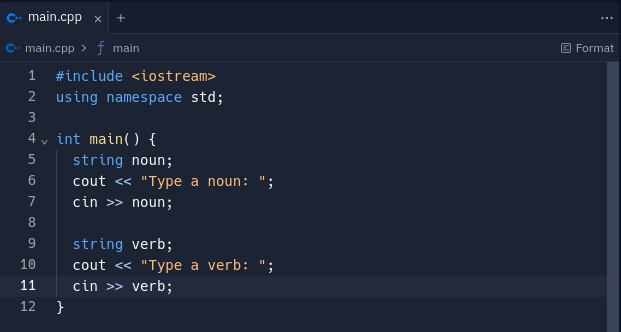
Now we are at the last step. This step will consist of a really big cout. The cout will contain a story, and you will replace some of the sections in the story with the variables you created. Here is the example story I will be making: cout << “Once there was a ” << noun << ” that ” << verb << ” really fast.” << endl; Make sure to separate the text and the variables with the << symbol and make a space in the text before your next variable. You can also write \n right before the first word to make the text go a line down in the console. See my example in the video below.